Python Programming
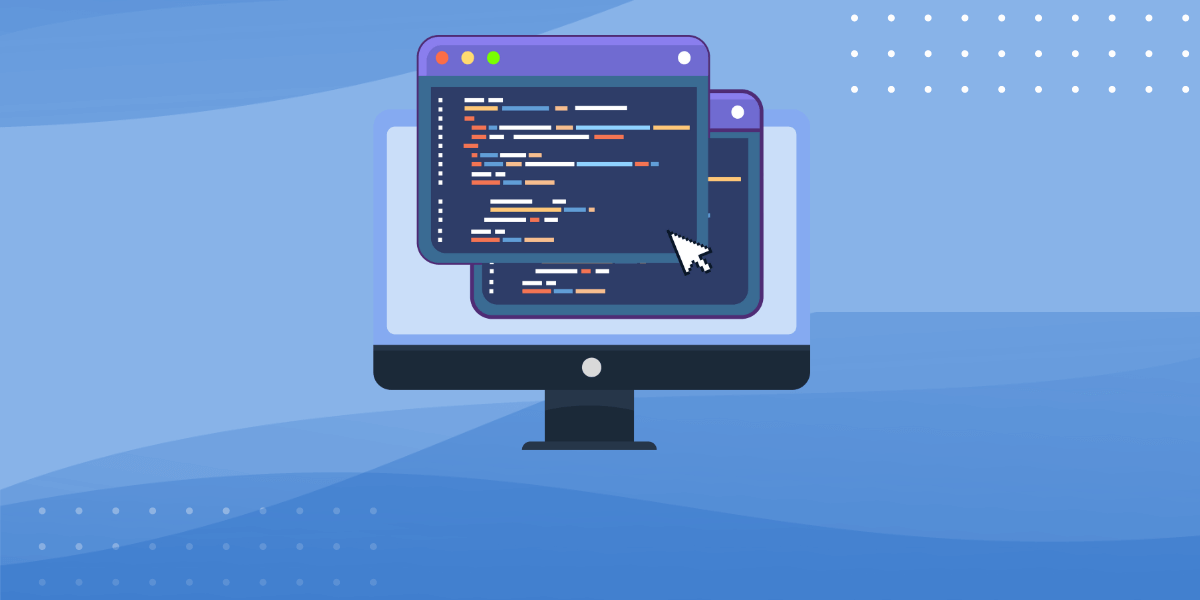
About Course
The IO Brain Python Programming Course is a comprehensive program designed to introduce students to the powerful world of Python programming. This course caters to both beginners and those looking to enhance their existing Python skills, offering a structured learning path that covers fundamental concepts as well as advanced programming techniques. Python's simplicity, readability, and versatility make it an ideal language for learners of all ages, and this course is tailored to equip participants with the skills needed to excel in various programming disciplines.
Course Objectives:
- Provide a solid foundation in Python programming, covering basic syntax, data types, and control structures.
- Enhance problem-solving skills through hands-on coding exercises and real-world projects.
- Develop proficiency in advanced Python concepts, including data structures, algorithms, and object-oriented programming.
- Introduce participants to practical applications of Python, such as web development, data analysis, and game development.
- Foster creativity and innovation through project-based learning and interactive exercises.
- Equip students with the skills to build, test, and debug Python applications efficiently.
Key Modules:
Python Basics:
- Introduction to Python:
- Overview of Python as a programming language.
- Understanding Python's role in modern software development.
- Setting up the Python environment and using the Python interpreter.
- Python Syntax and Data Types:
- Writing and executing Python code.
- Introduction to Python's indentation-based structure.
- Exploring basic data types: integers, floats, strings, and booleans.
- Working with variables, expressions, and statements.
- Control Structures:
- Understanding conditional statements (if, elif, else).
- Learning about loops (for, while) and their applications.
- Implementing basic error handling with try-except blocks.
- Functions and Modules:
- Defining and calling functions in Python.
- Understanding scope and lifetime of variables.
- Introduction to Python's standard library and importing modules.
- Working with Data Structures:
- Exploring lists, tuples, sets, and dictionaries.
- Understanding mutable vs. immutable data types.
- Performing operations on data structures (adding, removing, sorting).
- File Handling and I/O:
- Reading from and writing to files.
- Understanding file modes and working with different file formats.
- Basic input and output operations in Python.
Python Advanced:
- Object-Oriented Programming (OOP) in Python:
- Introduction to OOP concepts: classes, objects, inheritance, and polymorphism.
- Designing and implementing classes and objects.
- Understanding encapsulation and abstraction in Python.
- Advanced Data Structures and Algorithms:
- Exploring advanced data structures: stacks, queues, linked lists, and trees.
- Implementing sorting and searching algorithms.
- Introduction to algorithmic thinking and problem-solving strategies.
- Game Development with Python:
- Introduction to game development using Pygame.
- Creating basic game mechanics: player movement, collision detection, and scoring.
- Handling user input and managing game states.
- Data Analysis and Visualization:
- Introduction to data analysis libraries: NumPy, Pandas, and Matplotlib.
- Manipulating and analyzing datasets.
- Visualizing data trends and creating plots and charts.
- Graphical User Interface (GUI) Development:
- Introduction to GUI development using Tkinter.
- Designing interactive applications with buttons, menus, and graphics.
- Creating visually appealing and user-friendly interfaces.
- Collaborative Projects and Version Control:
- Working on collaborative Python projects.
- Introduction to version control with Git.
- Managing codebases and integrating team contributions.